OpenAPI provides a terrific UI to use for testing, learning and using your APIs. My team has built some APIs using Quarkus, and used their implementation of the SmallRye OpenAPI library. The problem I’ve run into is that the parameters of any given API call come out in alphabetical order, not the order specified in the code. Here’s a sample of the code with some OpenAPI metadata:
@GET
@Path("/range")
@Operation(summary = "Get a list of resources inclusive for a range of dates")
public RangeResult getRecordsByRange(
@Context UriInfo urlInfo,
@Parameter(description = "The starting date", required = true, example = "yyyy-MM-dd")
@QueryParam("startDate") String startDate,
@Parameter(description = "The ending date, inclusive of records generated on that day", required = true, example = "yyyy-MM-dd")
@QueryParam("endDate") String endDate,
@Parameter(description = "The current data page, using a zero-based index. Default value is zero (the first page)", required = false, example = "2")
@QueryParam("page") int page,
@Parameter(description = "The number of records per page (max 1000). Default value is 100.", required = false, example = "100")
@QueryParam("pageSize") int pageSize) throws ApiException {
}
Unfortunately, that code results in this:
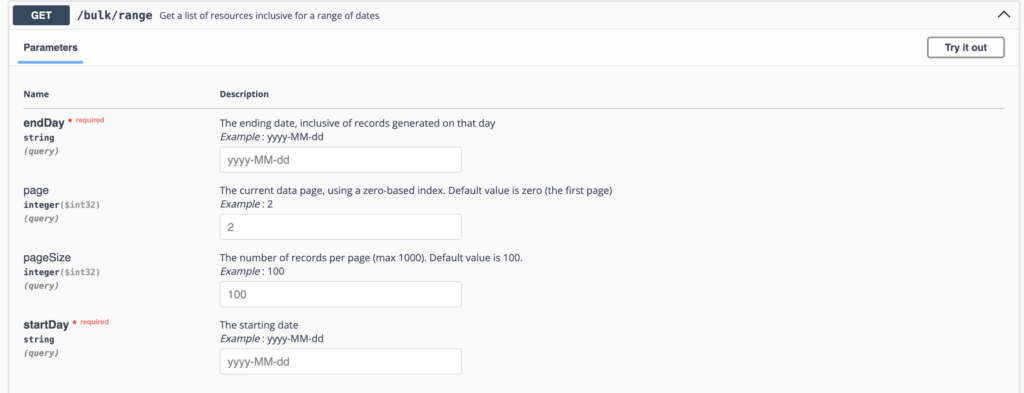
This doesn’t make sense to me for multiple reasons. First, the endDay parameter is before the startDay parameter. Second, those same parameters are required and they occur first and last. To make this more intuitive for the user, I’d want to group the required parameters at the beginning, and ensure that startDay comes before endDay.
The solution was suggested in a Github issue for Quarkus, I did not find the solution elsewhere, and it certainly was not intuitive. The answer was to not place the @Parameter
metadata inline with the function parameters (like all the OpenAPI examples I could find). By separating them from the function definition and placing them above the function, OpenAPI respected the order. I changed the code to this:
@GET @Path("/range") @Operation(summary = "Get a list of resources inclusive for a range of dates") @Parameter(description = "The starting date", required = true, example = "yyyy-MM-dd") @Parameter(description = "The ending date, inclusive of records generated on that day", required = true, example = "yyyy-MM-dd") @Parameter(description = "The current data page, using a zero-based index. Default value is zero (the first page)", required = false, example = "2") @Parameter(description = "The number of records per page (max 1000). Default value is 100.", required = false, example = "100") public RangeResult getRecordsByRange( @Context UriInfo urlInfo, @QueryParam("startDate") String startDate, @QueryParam("endDate") String endDate, @QueryParam("page") int page, @QueryParam("pageSize") int pageSize) throws ApiException { }
That change resulted in the below image, which is exactly what I wanted:
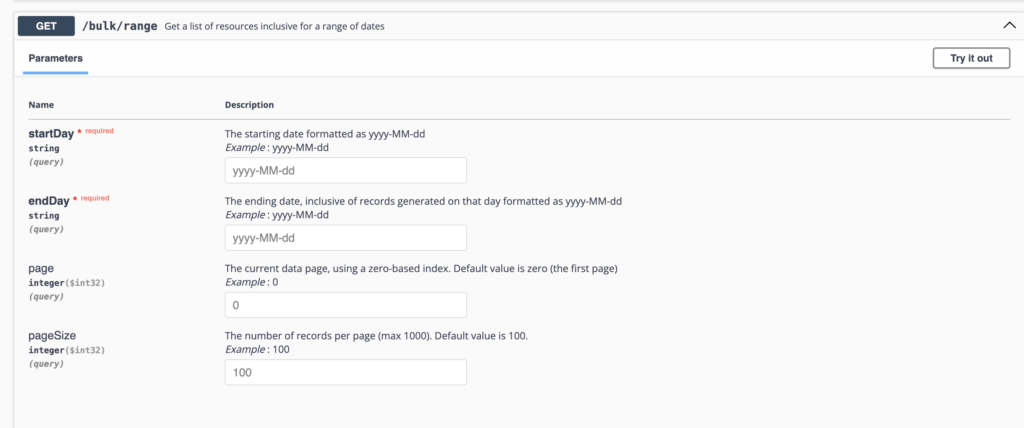